Are you tired of spending countless hours coding and debugging your web pages? Do you wish there was an easier way to create dynamic and interactive web pages? A free HTML JavaScript Editor might just be what you need. And why wouldn’t you? Almost 99% of all active websites use JavaScript! [1]
By the end of this article, you will have a comprehensive understanding of HTML JavaScript editor, how it compares to IDE, how to add it to your HTML pages, what some of your options are, and what you should consider before making your choice.
What is a JS editor?
First things first, let’s define what a JS editor is and why it’s important in creating websites.
A JavaScript editor, also known as a JS editor, is a specialized software tool that enables developers to write, edit, and manage JavaScript code efficiently. JavaScript is a programming language widely used for creating interactive and dynamic elements on websites. While HTML provides the structure and content of a web page, JavaScript adds functionality and interactivity to it. An HTML JavaScript editor, therefore, acts as a dedicated environment for writing and editing JavaScript code. Styling with JSE makes it easier for developers to create complex websites with different themes, fonts, text styles, and other formatting choices.
What does the JS editor really do?
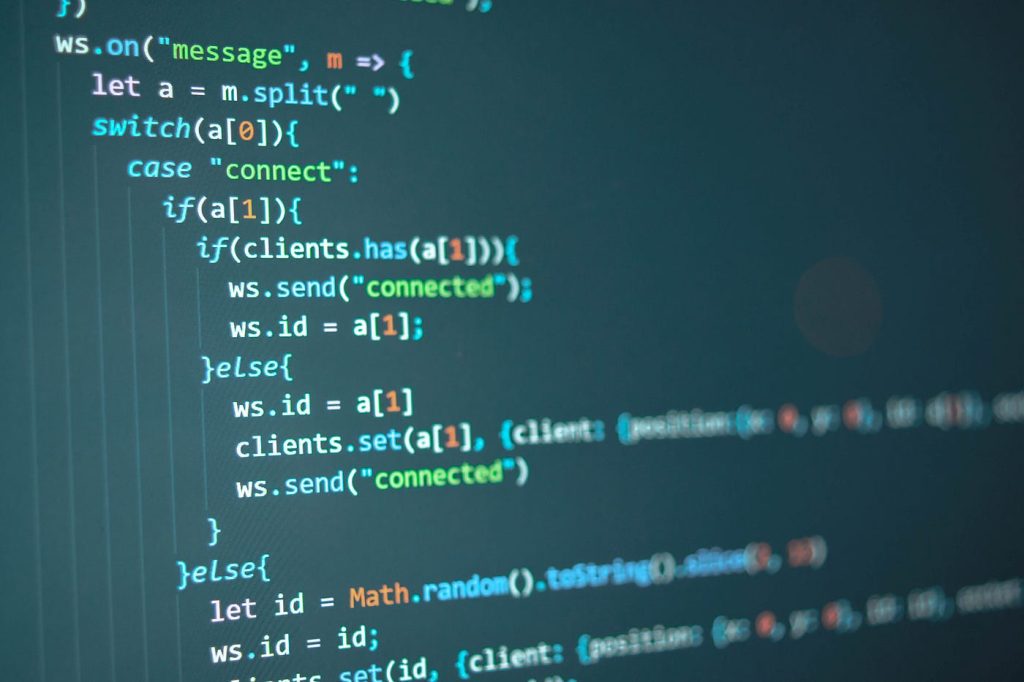
Now that we understand the basics let’s delve into the advantages and capabilities of a JS editor. An HTML JavaScript Editor:
- Provides a user-friendly interface with features like auto-indentation, code completion, and syntax highlighting. These features help developers write cleaner and error-free code, enhancing productivity and reducing debugging time.
- Simplifies the creation of complex web pages by providing ready-to-use code snippets and libraries. Instead of writing code from scratch, developers can leverage these resources to quickly add interactive elements, animations, and functionality to their websites.
- Includes built-in debugging tools, allowing developers to identify and fix errors efficiently.
- Enhances code organization and readability through features like code folding and indentation guides.
Difference between JSE and IDE
Understanding the distinction between a JavaScript editor and an Integrated Development Environment (IDE) is important. While both a JSE and an IDE are used for web development, the former focuses specifically on writing and editing JavaScript code, while the latter is a comprehensive software package that encompasses multiple programming languages.
The table below highlights the key differences between the two.
JSE | IDE |
Can only write and edit code | Can test and preview code projects |
Features tailored to JavaScript’s syntax and conventions | Features like version control integration, project management, code compiling, and extensive debugging capabilities |
As easy as learning to code in JavaScript | More complex and take longer to learn |
More suitable for smaller web development tasks that primarily involve JavaScript | Generally preferred for large-scale projects that involve multiple languages or complex frameworks |
How do you add JSE to your page?
Adding a JavaScript editor to your HTML page is a straightforward process. Here is a step-by-step guide to help you integrate a JavaScript editor into your web development workflow:
Step 1: Choose a JavaScript editor
Evaluate the available JavaScript editors based on your requirements and preferences. Consider factors such as ease of use, community support, and compatibility with your development environment. For the purpose of this guide, we will use Froala Editor as an example. However, keep in mind that the process may vary slightly depending on the specific editor you choose.
Step 2: Download the JavaScript editor
Visit the official website of your chosen HTML JavaScript editor and download the appropriate version for your operating system. Ensure you have the necessary JavaScript and CSS files required for the editor.
Step 3: Install the JavaScript editor
Run the installer file and follow the on-screen instructions to install the JavaScript editor on your machine.
Step 4: Launch the JavaScript editor
Once the installation is complete, open the JavaScript editor to begin writing and editing your JavaScript code.
Step 5: Create the HTML Structure
Start by creating the basic HTML structure for your page. This typically includes the <!DOCTYPE html> declaration, <html> tags, <head> section, and <body> tags. Make sure to link the CSS and JavaScript files appropriately.
<!DOCTYPE html>
<html>
<head>
<link rel="stylesheet" href="path/to/editor.css">
<script src="path/to/editor.js"></script>
</head>
<body>
<!-- Your HTML content goes here -->
</body>
</html>
Step 6: Create a TextArea Element
Next, add a <textarea> element to your HTML file. This element will serve as the container for the JS Editor.
<textarea id="editor"></textarea>
Step 7: Initialize the JS Editor
To initialize the JS Editor, use JavaScript code within a <script> tag. In this example, we will initialize the Froala Editor on the previously created <textarea> element with the id “editor”.
<script>
new FroalaEditor('#editor');
</script>
Step 8: Customize Editor Options (Optional)
You can customize the JS Editor by specifying additional options during initialization. These options allow you to control various aspects of the editor’s behavior, such as toolbar buttons, plugins, and styling.
<script>
new FroalaEditor('#editor', {
toolbarButtons: ['bold', 'italic', 'underline', 'paragraphFormat', 'insertLink'],
pluginsEnabled: ['codeView'],
// Additional options...
});
</script>
Step 9: Save the HTML File
Save the HTML file with a descriptive name, such as “index.html”, and open it in a web browser. You should now see the JS Editor integrated into your HTML page.
Step 10: Test your JavaScript code
Load your HTML page in a web browser to test the functionality of your JavaScript code. Use the browser’s developer tools to debug any issues and make necessary adjustments in the JavaScript editor.
You can now utilize the features and capabilities of the editor to create and edit JavaScript code directly within your web application. Remember to refer to the documentation and instructions provided by the specific JS Editor you choose for any additional customization or configuration options.
Creating text editor using JSE and HTML
One practical application of a JavaScript editor is creating a text editor within an HTML page. This allows users to write and edit text directly on a website, offering a convenient way to take notes, create forum posts, write articles, leave comments, or provide input.
Let’s dive into the step-by-step process of developing a text editor and explore an example using Froala Editor. We’ll also discuss styling options to make the text editor visually appealing.
Step 1: Set up the HTML structure
Begin by creating the basic HTML structure for your text editor. This typically involves creating a <textarea> element where users can input and edit text.
<textarea id="myEditor"></textarea>
Step 2: Add JavaScript code
Use your JavaScript editor to write the necessary JavaScript code to handle text editing functionalities. This may include features such as text formatting, word count, spell-checking, and saving the text to a file or a database.
First, include the necessary JavaScript and CSS files for Froala Editor:
<link rel="stylesheet" href="path/to/froala_editor.css">
<script src="path/to/froala_editor.js"></script>
Then, initialize the Froala Editor on the <textarea> element:
<script>
new FroalaEditor('#myEditor');
</script>
Step 3: Style the text editor
Utilize CSS to enhance the appearance of your text editor. You can customize the font, color, background, and other visual aspects to match your website’s design. Here’s an example of styling the Froala Editor:
/* Custom styling for the text editor */
#myEditor {
width: 100%;
height: 300px;
font-family: Arial, sans-serif;
font-size: 14px;
color: #333;
background-color: #fff;
border: 1px solid #ccc;
border-radius: 4px;
}
Step 4: Test and refine
Load the HTML page with your text editor in a web browser and thoroughly test its functionality. Make any necessary refinements or bug fixes using your JavaScript editor. Following from the example above, remember to refer to the Froala Editor documentation for advanced customization options and additional features.
Best JavaScript editor choices
When selecting a JavaScript editor, it’s essential to consider your specific requirements and the features different editors offer. JS editor features like functionality, ease of use, debugging capabilities, customer support, user-friendliness, and customizability are some factors that may influence your decision.
Here are some popular JavaScript editors, along with their key features to help you make an informed decision:
- Froala: Froala is known for its extremely user-friendly and customizable interface. It features a modern look and feel, and it packs a punch for how lightweight it is. It also works with all major frameworks and programming languages.
Best for: Easy and lightweight platform integration
Not recommended for: Beginners looking for a cheap solution - Visual Studio Code (VS Code): VS Code is a highly customizable and feature-rich JavaScript editor. It offers built-in support for debugging, Git integration, intelligent code completion, and a vast library of extensions to enhance productivity.
Best for: Customizing to suit your specifications
Not recommended for: Efficient battery consumption as it can be power-hungry - Sublime Text: Sublime Text is known for its speed and simplicity. It provides a distraction-free writing environment, powerful search-and-replace functionality, and a rich selection of plugins.
Best for: Reading large amounts of code with tag and syntax highlighting.
Not recommended for: Git integration - Atom: Atom is an open-source JavaScript editor developed by GitHub. It offers a clean and intuitive user interface, extensive customization options, and a large community that contributes to its continuous improvement.
Best for: Git integration and cross-platform integration
Not recommended for: Fast code execution as it can sometimes be very slow
Things to consider in choosing JavaScript editors
Here are key factors that you should consider when choosing between the various JS editors on the market.
- Features and Functionality: Look for features such as code autocompletion, syntax highlighting, error checking, code navigation, intelligent suggestions, debugging tools, version control integration, and support for plugins or extensions.
- User Interface and User Experience: A clean and intuitive user interface can greatly impact your productivity and overall experience while coding. Look for an editor with a well-designed interface that allows for easy navigation, customization, and readability.
- Extensions and Customization: Consider the extensibility of the JavaScript editor. Look for an editor that supports popular extensions or has an active community contributing to its extension library.
- Community and Support: Evaluate the community around the JavaScript editor. Look for an editor that has a strong community presence, where you can find helpful resources, tutorials, and forums for assistance.
- Integration with Development Tools and Frameworks: Consider how well the JavaScript editor integrates with other tools and frameworks that are essential to your development workflow. Look for compatibility with version control systems (e.g., Git), package managers (e.g., npm, Yarn), and popular JavaScript frameworks (e.g., React, Angular, Vue.js).
- Performance and Speed: Assess how responsive the editor is when handling large codebases, complex projects, or resource-intensive tasks. Look for a fast and efficient editor that ensures that you can write, modify, and test your code without significant lag or delays.
- Cost and Licensing: Some editors are open-source and freely available, while others may have a commercial or subscription-based model. Consider your budget and project requirements when choosing between free and paid options.
JavaScript increases efficiency
One of the primary advantages of using a JavaScript editor in your HTML development process is the increase in efficiency it brings. Quickly prototyping and developing interactive web elements, simplified debugging, code reusability, enhanced collaboration, and integration with libraries and frameworks are just some of the ways in which a JS editor makes your development process easier.
You can also improve productivity with JavaScript Editor by making use of real-time editing options, tracking changes, generating PDFs, embedding rich text and multimedia files, and using grammar and spell-check.
Conclusion
An HTML JavaScript editor is a powerful tool that can greatly enhance your web development experience. It allows you to easily create complex web pages, saving time and effort in the process. When choosing a JavaScript editor, consider factors like the required features, user interface, performance, extensibility, community support, and integration capabilities. By leveraging the advantages of a JavaScript editor, you can increase your efficiency, simplify debugging, and take your HTML Javascript development to the next level.
FAQs
- What editor can run JavaScript code?
Some of the best JavaScript code editors include Froala, Visual Studio Code, Atom, and Sublime Text.
- How to make HTML code editor in JavaScript?
First you would set up the HTML structure. Then, add JavaScript code to handle text editing functionalities. Next, you could utilize CSS to enhance the appearance of your text editor. Finally, test the HTML page with your text editor in a web browser.
- How to write JavaScript code in HTML?
In HTML, JavaScript code is written within <script> and </script> tags.
[1] https://w3techs.com/technologies/details/cp-javascript