What is Angular?
Frameworks provide a consistent structure that ensures developers do not have to rebuild code from scratch, significantly improving web development efficiency and performance. They offer various extra features you can add to your software without any effort.
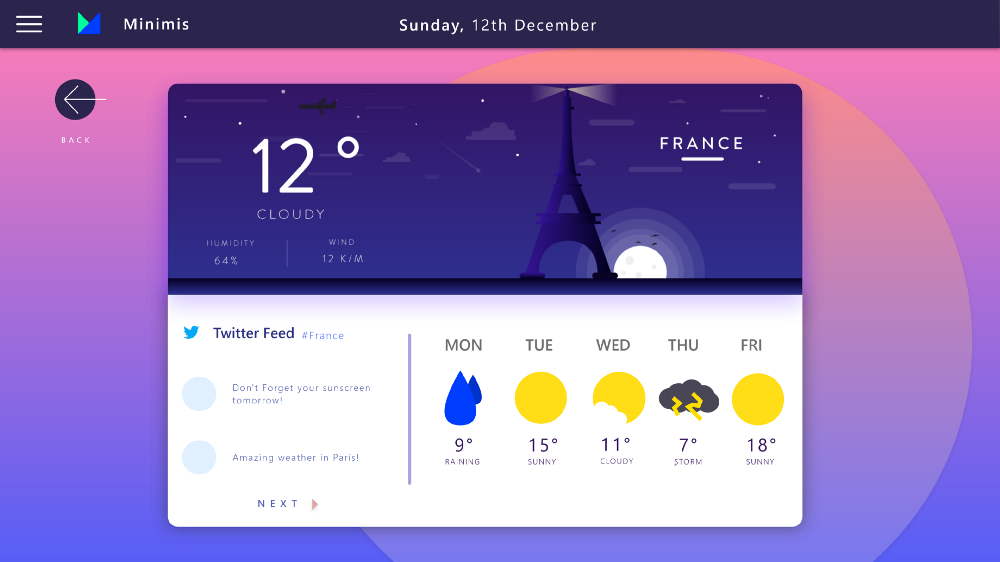
Angular is a framework written in TypeScript. You can use it to build single-page client applications with TypeScript and HTML. The framework is component-based, allowing you to build scalable web applications.
Angular implements functionality as TypeScript libraries that you can import into applications. It includes a collection of integrated libraries with various features, such as routing, client-server communication, file upload, and forms management. Angular also provides tools for developing, building, testing, updating code, and uploading files.
What is Authentication?
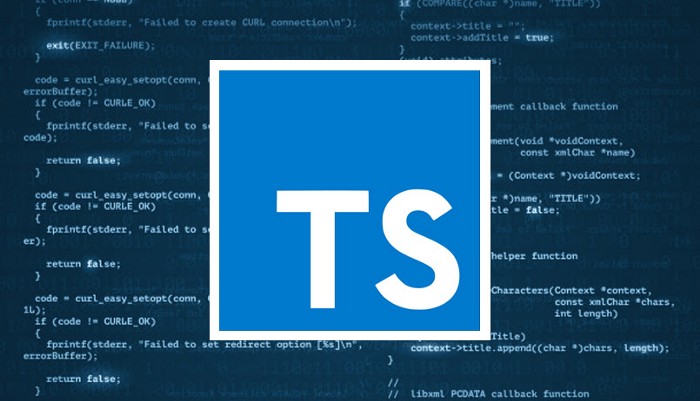
Authentication is a basic access control implemented to protect systems, applications, data, and networks. Authentication technology requires users to prove their identity by providing various factors, like usernames and passwords, IDs, fingerprints, irises, and more. Once the computer system recognizes these authentication credentials, it recognizes the user as a legitimate one.
Authentication is a foundation of network security, and is becoming more important. Many organizations are transitioning to a Zero Trust security model, in which all connections, whether from external parties or internal users within an organization’s network, must be authenticated and verified.
Authentication in Angular With Auth Guards and JWT
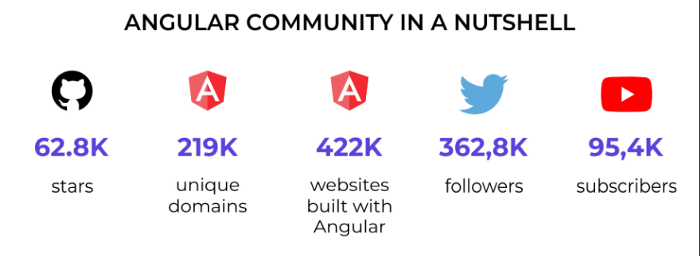
Angular allows controlling access to parts of an application by making it authentication or permission-based through Route Guards. Route Guards are interfaces that tell an Angular application router whether or not to allow a request to access a specific route.
Create Authentication Service
User authentication in Angular is built using the canActivate lifecycle event. It returns true if the user can access a page and false otherwise. This tutorial uses JSON web tokens for user authentication.
To create an authentication service in Angular:
Use the following command to generate the authentication service:
ng g service demoAuth
This command will create an AuthGuard called demoAuth and a file with the following AuthGuard boilerplate code in the services folder.
import { Injectable } from '@angular/core';
@Injectable({
providedIn: 'root'
})
export class AuthService {
constructor() { }
isLoggedIn() {
const token = localStorage.getItem('token'); // get token from local storage
const payload = atob(token.split('.')[1]); // decode payload of token
const parsedPayload = JSON.parse(payload); // convert payload into an Object
return parsedPayload.exp > Date.now() / 1000; // check if token is expired
}
}
The isLoggedIn() function checks the JSON token in local storage and checks validity for user authentication.
Create and Enforce Route Guard
To create a Route Guard and implement it:
- Use the following command to create the guard:
ng g guard demoAuth
This command generates a file named demoAuth.guard.ts. In addition, it implements the CanActivate interface:
import { Injectable } from '@angular/core';
import { CanActivate, ActivatedRouteSnapshot, RouterStateSnapshot, Router } from '@angular/router';
import { Observable } from 'rxjs';
import { AuthService } from './demoAuth.service';
@Injectable({
providedIn: 'root'
})
export class AuthGuard implements CanActivate {
constructor(private authService: AuthService, private router: Router) {}
canActivate(
next: ActivatedRouteSnapshot,
state: RouterStateSnapshot): Observable<boolean> | Promise<boolean> | boolean {
if (!this.authService.isLoggedIn()) {
this.router.navigate(['/login']); // go to login if not authenticated
return false;
}
return true;
}
}
The canActivate() method uses AuthGuard and its JSON validating functionality to check if a user is logged in or out.
- Use the following code to put the route guard to use through canActivate():
import { NgModule } from '@angular/core';
import { Routes, RouterModule } from '@angular/router';
import { DemoAppHomeComponent } from './home/home.component';
import { DemoAppLoginComponent } from './login/login.component';
import { AuthGuard } from './demoAuth.guard';
const routes: Routes = [
{ path: '', redirectTo: '/home', pathMatch: 'full' },
{ path: 'demoLogin', component: DemoAppLoginComponent },
{ path: 'demoHome', component: DemoAppHomeComponent,
canActivate: [AuthGuard],
},
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule]
})
export class AppRoutingModule { }
Now, the user cannot access the homepage unless they are logged in and authenticated.
Conclusion
In this article, I showed how the Angular framework provides built in authentication, and showed how to implement it with Guard Rails, the Angular mechanism for determining if a user should be allowed to view a certain application path or not. The process involves:
- Generating the authentication service
- Creating an implementing a Route Guard
- Putting the route guard to use through the canActivate() function
It’s a bit complex, but once you get the hang of it, you can implement authentication in Angular applications seamlessly and securely.